Enhancing Python Code Reliability with Mypy Type Checker
Written on
Chapter 1: Understanding Mypy
Mypy serves as a static type checker for Python, enabling developers to use type hints in their code. By doing so, it ensures that the code adheres to these hints during execution. This process aids in identifying bugs and enhances the overall reliability of Python programs by flagging type errors prior to execution.
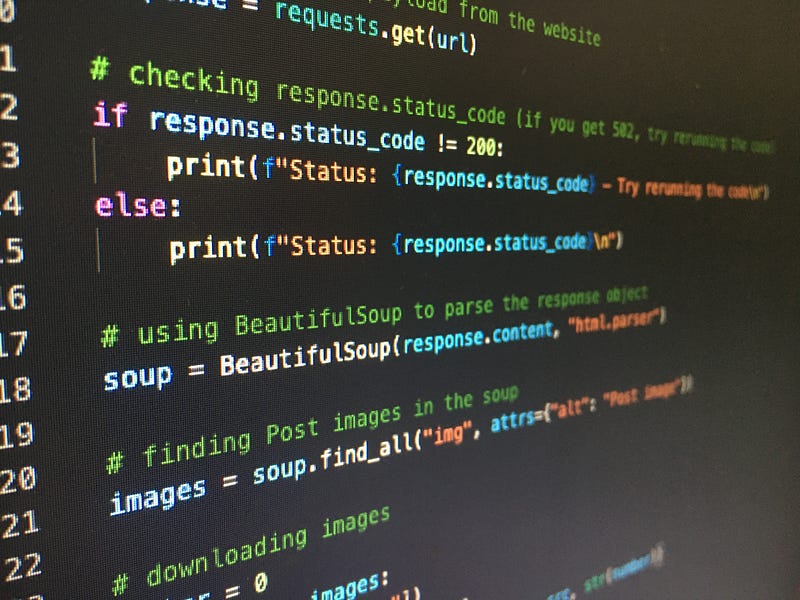
Photo by Artturi Jalli on Unsplash
To begin utilizing Mypy, installation can be accomplished via the command: pip install mypy. After installation, you can execute Mypy on your Python script by typing mypy my_script.py in the terminal. Mypy will analyze your code for type errors and report any discrepancies it encounters.
Section 1.1: Simple Mypy Usage Example
Let’s examine a straightforward instance of how to apply Mypy with a Python function:
def greet(name: str) -> str:
return f'Hello, {name}!'
print(greet('Alice'))
print(greet(123))
In this scenario, the greet function is defined to accept a parameter name and return a string. We specify name with the type hint str, indicating that it should be a string. The function's return type is also annotated as str, clarifying that it should yield a string output.
When this code is processed through Mypy, it will flag an error on the second greet call since an integer (123) is provided instead of a string. This functionality is essential for identifying issues in the code before they escalate into runtime errors.
Section 1.2: Advanced Type Hints
Beyond basic type hints, Mypy accommodates more complex features like generics and union types. Consider the following example:
from typing import List, Union
def sort_items(items: List[Union[int, str]]) -> List[Union[int, str]]:
return sorted(items)
print(sort_items([1, 2, 3]))
print(sort_items(['a', 'b', 'c']))
print(sort_items([1, 'a', 2, 'b']))
Here, the sort_items function is designed to accept a list of items and return a sorted version of that list. We annotate the input parameter items with the type hint List[Union[int, str]], indicating that it can contain either integers or strings. The return type is similarly annotated.
When running this code through Mypy, no errors will surface because all calls to sort_items provide lists that align with the specified type hints.
Chapter 2: Mypy’s Compatibility Checks
This video, Static type checking with Mypy — Perfect Python, elucidates the significance of Mypy in enhancing Python code quality through static type checking.
In this second video, Introduction to Python Typing + Mypy (Beginner - Intermediate), Anthony provides an insightful overview of how to effectively utilize typing with Mypy.
Mypy is also capable of verifying code compatibility across various Python versions. For instance, to ensure compatibility with both Python 3.8 and 3.9, you can run:
mypy --pyversion 3.8 my_script.py
mypy --pyversion 3.9 my_script.py
This feature proves invaluable for confirming that your code is functional across a diverse range of Python environments.
In conclusion, Mypy stands out as a robust tool for boosting the reliability and maintainability of Python code. By incorporating type hints, developers can identify type errors early, enhancing compatibility with different Python versions. While it does not replace traditional testing, Mypy acts as a safeguard against common pitfalls, making the codebase more comprehensible and manageable.
One crucial distinction regarding Mypy is that it functions as a static type checker—not a runtime type checker. This implies that it evaluates the types of variables and expressions at compile time rather than at runtime. Consequently, Mypy can only identify type errors that it can deduce from the type hints provided in your code and may miss errors arising from runtime computations or external inputs.
Despite these constraints, Mypy is an exceptionally beneficial tool for elevating the quality and dependability of Python code. It enjoys widespread usage within the Python community and has a proven track record of assisting developers in detecting and rectifying type-related issues. If you haven’t yet integrated Mypy into your Python projects, we highly encourage you to explore its capabilities.
For more insights, visit PlainEnglish.io.
Stay updated with our free weekly newsletter and follow us on Twitter, LinkedIn, YouTube, and Discord. If you’re looking to enhance awareness and adoption for your tech startup, consider checking out Circuit.