Create a Simple Quote of the Day App Using Vue 3 and JavaScript
Written on
Chapter 1: Introduction to Vue 3
Vue 3 is the most recent iteration of the user-friendly Vue JavaScript framework designed for developing front-end applications. In this guide, we will explore how to build a Quote of the Day application using Vue 3 and JavaScript.
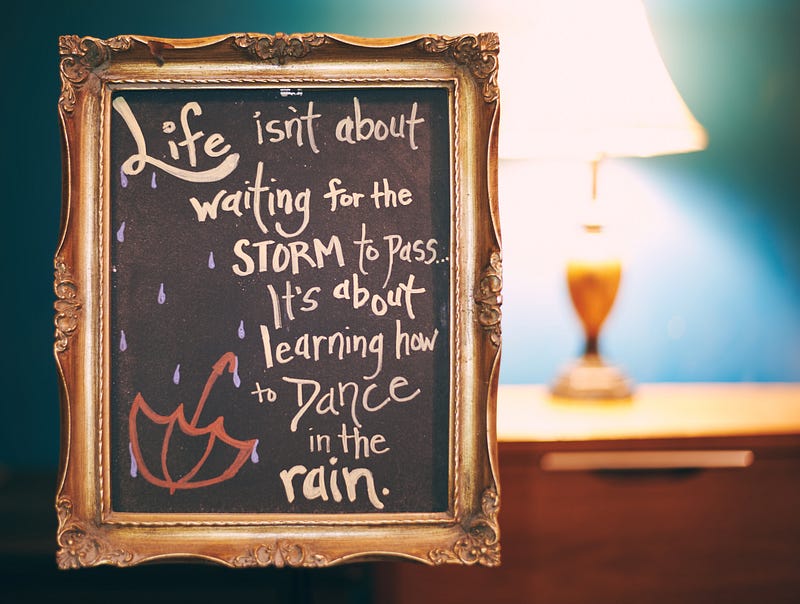
Chapter 2: Setting Up the Project
To initiate the Vue project, we utilize the Vue CLI. To install it, execute the following command using NPM:
npm install -g @vue/cli
Alternatively, you can use Yarn:
yarn global add @vue/cli
Next, create the project with the following command:
vue create quote-of-the-day-app
Choose the default settings for a smooth setup.
Chapter 3: Building the Quote of the Day App
We can construct our Quote of the Day application by implementing the following code:
<template>
<button @click="getRandomQuote">Get Quote</button>
<p>{{ quoteOfTheDay }}</p>
</template>
<script>
export default {
name: "App",
data() {
return {
quotes: [],
quoteOfTheDay: "",
};
},
methods: {
async getQuotes() {
const quotes = await res.json();
this.quotes = quotes;
},
getRandomQuote() {
const index = Math.floor(Math.random() * this.quotes.length);
const quoteOfTheDay = this.quotes[index];
this.quoteOfTheDay = quoteOfTheDay.text;
},
},
async beforeMount() {
await this.getQuotes();
this.getRandomQuote();
},
};
</script>
In this component structure, the data method returns an object containing reactive properties: quotes (an array of quotes) and quoteOfTheDay (a randomly selected quote).
The methods section includes:
- getQuotes: Fetches quotes from the specified URL and assigns the resulting JSON array to this.quotes.
- getRandomQuote: Randomly selects a quote from the quotes array and assigns it to quoteOfTheDay.
The beforeMount lifecycle hook ensures that the quotes are fetched and a random quote is selected when the component loads.
In the template, we present a button that triggers the getRandomQuote function, displaying the result in a paragraph element.
The first video titled "Build a Quote Generator in VueJS | A Vue JS Beginner Project" provides a hands-on approach to building a similar application.
Chapter 4: Conclusion
In summary, creating a Quote of the Day application with Vue 3 and JavaScript is straightforward and efficient.
For a more interactive experience, check out the second video, "Live Coding Project: Create a Random Quote Machine using Vue.js - with Gwen Faraday", which walks you through the process in real-time.