Unlocking the Power of Firebase Cloud Functions in Flutter
Written on
Getting Started with Firebase Cloud Functions
In this guide, I will walk you through the process of writing, deploying, and invoking a cloud function using Firebase from your Flutter application.
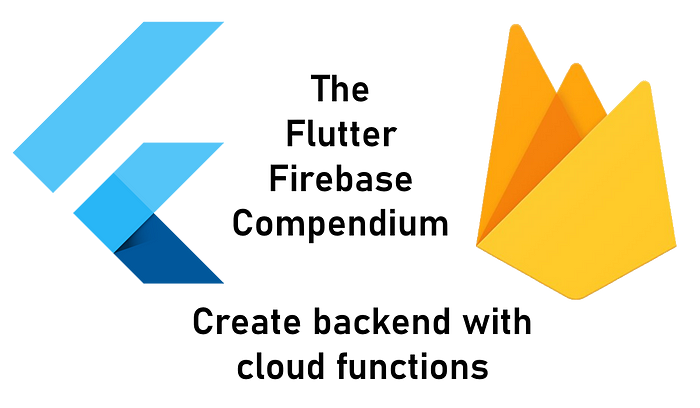
Harnessing External Processing Power
Firebase Cloud Functions is a Google service that allows you to run code in the cloud, offering significant advantages in scalability. With Firebase, your app can effectively handle a large number of users without performance issues. This article will cover several key topics:
๐น Configuring Firebase with your app
๐น Initializing cloud functions
๐น Crafting a cloud function
๐น Deploying a cloud function
๐น Invoking a cloud function
๐น Using functions as HTTP request endpoints
๐น Scheduling functions
๐น Selecting regions
๐น Logging
Want to dive deeper? Check out my ebook for extensive guides on building Flutter apps with Firebase, available on Gumroad!
Configuring Firebase and Your App
To get started, you need to set up Firebase and connect it to your application. For detailed instructions on this process, please refer to the linked article.
Next, install the Firebase Cloud Functions package by adding it to your pubspec.yaml file or via the command line. If you need more guidance, consult the installation manual or the previous article.
With the setup complete, you can begin writing your functions! ๐
Initializing Cloud Functions
Log in to Firebase by executing the command firebase login. Next, run firebase init functions from your project's root directory. The tool will prompt you with several questions that you'll need to answer, such as:
๐น Are you ready to continue? โ Yes
๐น Please select an option: โ Use an existing project
๐น What language do you want to use for Cloud Functions? โ JavaScript
๐น Would you like to use ESLint to catch potential errors and enforce style? โ No
๐น Do you want to install dependencies with npm now? โ Yes
After completing these steps, a new functions folder will appear in your project structure.
Writing a Cloud Function
Now, let's write some code that will run in the cloud. For our example, we will create a function that takes a string as input and converts it to uppercase. This simple code will illustrate what you can achieve.
Open index.js in the functions folder and include the following code:
exports.toUpperCase = functions.https.onCall((data, context) => {
return data.text.toUpperCase();
});
Here, we define a callable method called toUpperCase with two parameters. The data parameter contains the string we want to modify, and the second parameter is a CallableContext, which is not utilized in this example. We simply use JavaScript's built-in toUpperCase method and return the result. It's that straightforward! ๐
๐ก Ensure your code is idempotent! This means it should produce the same output even when called multiple times, which simplifies the process of retrying failed executions.
๐ก You can opt for TypeScript as your programming language. Just select "TypeScript" during the initialization prompt instead of "JavaScript." Currently, these are the only supported languages.
Deploying a Cloud Function
Deployment is a breeze! Simply run the command firebase deploy --only functions and wait for the process to complete. It may take up to five minutes for your dashboard to refresh and display the updated information about logs and function usage.
Calling a Cloud Function
A callable function can be invoked using the method functions.https.onCall((data, context) => {...});. The Firebase dashboard does not differentiate between callable functions and HTTP endpoints; both triggers are categorized as "Request."
To call a function named toUpperCase(), refer to the following code example:
const result = await firebase.functions().httpsCallable('toUpperCase')({ text: "hello" });
Defining an HTTP Request Endpoint
Using the syntax functions.https.onRequest((request, response) => {...});, you can create an HTTP endpoint that can be called by other web services. This allows you to define interfaces for third-party applications to interact with your data or logic. You do not necessarily need the Firebase package to call endpoints, but you will require something to create requests, such as the http or Dio packages. Hereโs an example:
๐ก Remember to replace the link to the function with your deployed version!
For those unfamiliar with sending HTTP requests in Flutter, I have an article with demo code that could be beneficial.
โ By default, an HTTP endpoint is accessible to anyone. Be sure to implement security measures like authorization tokens. For an official example of securing your endpoints, click here.
Scheduling a Function
You can also set a function to run on a schedule. The interval can be specified using the App Engine cron.yaml syntax or the traditional Unix crontab syntax. Hereโs an example that executes a function every 10 minutes:
exports.scheduledFunction = functions.pubsub.schedule('every 10 minutes').onRun((context) => {
console.log('This will be run every 10 minutes!');
});
For a list of supported timezones, click here.
Selecting Regions
Firebase offers different regions that impact both pricing and latency. You can specify your preferred region using the region() method with a valid region name as an argument. For instance: functions.region("europe-west3"). I recommend choosing the nearest region to your location and you can also specify multiple regions separated by commas.
Logging
To monitor function executions, you can utilize logging. The output will be visible in your Firebase Functions dashboard.
const functions = require("firebase-functions");
functions.logger.log("This is a log:", someObj);
functions.logger.info("This is an info message:", someObj);
functions.logger.warn("This is a warning:", someObj);
functions.logger.error("This is an error message:", someObj);
For additional details on logging, refer to the official documentation.
Callable Functions vs. HTTP Functions
Callable functions and HTTP functions are quite similar. A callable function is essentially an HTTP function with special request parameters. The main distinction lies in that authentication tokens for Firebase and Firebase Cloud Messaging, along with App Check tokens, are automatically included in the request for callable functions if available. Additionally, callable functions validate these tokens by default, meaning you gain authentication features without extra work.
On the other hand, HTTP functions do not come with built-in access control, so you will need to implement security measures on your own. However, you can still invoke a callable function via an HTTP request by supplying the correct data.
โ Use callable functions if your app is the sole consumer.
โ Opt for HTTP functions if you have third-party consumers (like a public REST API) but ensure you implement access control and other security measures.
Conclusion
Firebase Cloud Functions present an efficient way to leverage external processing power in your Flutter application. Hereโs a brief demo video showcasing the source code.
You can find the complete example source code on my GitHub page.
This article is part of the Flutter Firebase Compendium, which contains numerous tutorials and guides on how to maximize Firebase's capabilities in Flutter applications.
Enhance your Firebase knowledge for Flutter developers with my ebooks!